First things first. Here we won’t be discussing mocking of Java enum or final class using PowerMock framework. That is already discussed in tons of places & one of the main reason why people tend to use PowerMock.
Here we will be discussing mocking of enum or final classes with Mockito framework only. The feature has been there from Mockito version 2.1.0. But it is not that well known & requires explicit configuration to enable this feature.
To use this feature, use Mockito version 2.1.0 or higher.
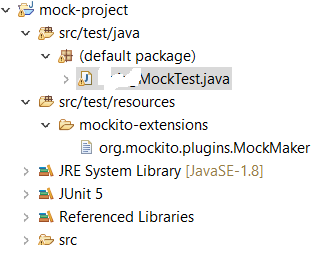
To explicitly enable the settings, we need to create mockito-extension directory under src/test/resources/ path. Then we should create a new file named org.mockito.plugins.MockMaker under mockito-extension directory & add below line there:
mock-maker-inline
This feature is turned off by default as it uses different mocking mechanism than the standard one & Mockito folks are still collecting feedback on that. Anyways that’s all there to configure.
Without this configuration, if you try to run below test case, you will get error saying
org.mockito.exceptions.base.MockitoException:
Cannot mock/spy class EnumMockTest$TEST_ENUM
Mockito cannot mock/spy because :
- final class
import static org.mockito.Mockito.mock;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.junit.platform.runner.JUnitPlatform;
import org.junit.runner.RunWith;
import org.mockito.junit.jupiter.MockitoExtension;
@ExtendWith(MockitoExtension.class)
@RunWith(JUnitPlatform.class)
public class EnumMockTest {
public static enum TEST_ENUM{
VAL1;
}
@Test
public void enumMockTest() {
TEST_ENUM mockStr = mock(TEST_ENUM.class);
}
}
But your test case will pass & you will see a Junit green bar once you turn on mock maker feature as mentioned above. This way you can mock Java enum or a final class with Junit & Mockito. You don’t need any other additional framework.